[LeetCode] 11.Implement strStr()
leetcode.com/problems/implement-strstr/
Implement strStr() - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
1. Problem
Implement strStr().
Return the index of the first occurrence of needle in haystack, or -1 if needle is not part of haystack.
Clarification:
What should we return when needle is an empty string? This is a great question to ask during an interview.
For the purpose of this problem, we will return 0 when needle is an empty string. This is consistent to C's strstr() and Java's indexOf().
Example 1:
Input: haystack = "hello", needle = "ll"
Output: 2
Example 2:
Input: haystack = "aaaaa", needle = "bba"
Output: -1
Example 3:
Input: haystack = "", needle = ""
Output: 0
Constraints:
- 0 <= haystack.length, needle.length <= 5 * 104
- haystack and needle consist of only lower-case English characters.
2. Code
package leetCode;
public class ImplementStrstr {
public static void main(String[] args) {
String haystack = "mississippi";
String needle = "pi";
strStr(haystack, needle);
}
public static int strStr(String haystack, String needle) {
if(needle.equals(0)) {
return 0;
}
for(int i = 0; i < haystack.length() - needle.length() +1; i++) {
boolean include = true;
for(int j = 0; j < needle.length(); j++) {
//System.out.println(haystack.charAt(i+j) + " " + needle.charAt(j));
if(haystack.charAt(i+j) != needle.charAt(j)) {
include = false;
break;
}
}
if(include) {
return i;
}
}
return -1;
}
}
3. Report
equals 메소드는 비교하고자 하는 대상의 내용 자체를 비교하지만, == 연산자는 비교하고자 하는 대상의 주소값을 비교합니다.
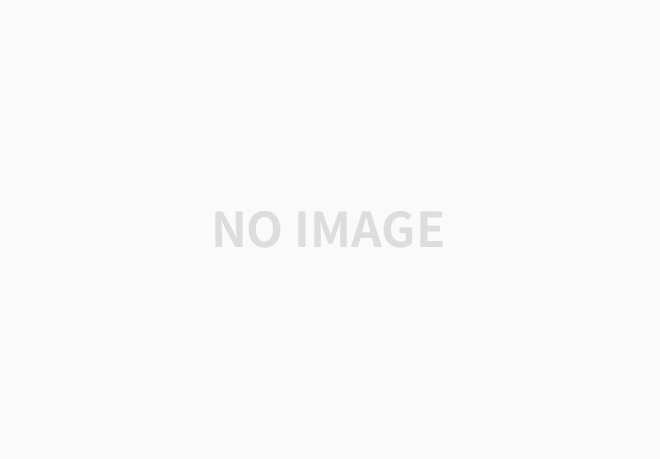
이 문제는 string.indexOf(string)을 구현하는 것과 같다
haystack.indexOf(needle)을 써도 제출이 가능하다 ㅎ
'Algorithm > 문제' 카테고리의 다른 글
[LeetCode] 13.Plus One (0) | 2021.01.17 |
---|---|
[LeetCode] 12.Length of Last Word (0) | 2021.01.14 |
[LeetCode] 10.Remove Duplicates from Sorted ArrayⅡ (0) | 2021.01.04 |
[LeetCode] 9.Remove Duplicates from Sorted Array (0) | 2021.01.04 |
[LeetCode] 8.Remove Element (0) | 2021.01.04 |